Objective:
This problem is a basic exercise in iterating through an array and summing its elements, which helps in understanding fundamental array operations and basic input/output in programming. Let’s complete the simple array sum function by understanding the problem.
Simple Array Sum Problem Statement:
You need to write a function that takes an array of integers as input and returns the sum of its elements.
Input Format:
- The first line contains an integer
n
, the number of elements in the array. - The second line contains
n
space-separated integers representing the elements of the array.
Output Format:
- A single integer represents the sum of the elements in the array.
Example 1:
- Input:
n = 6
array = [1, 2, 3, 4, 10, 11]
- Output:
31
- Explanation: The sum of the elements 1, 2, 3, 4, 10, and 11 is 31.
Example 2:
- Input:
n = 4
array = [1, 2, 3, 4]
- Output:
10
- Explanation: The sum of the elements 1, 2, 3, and 4 is 10.
Example 3:
- Input:
n = 3
array = [5, 5, 5]
- Output:
15
- Explanation: The sum of the elements 5, 5, and 5 is 15.
Steps to Solve:
- Read the Input:
- Read the integer
n
(number of elements in the array). - Read the
n
space-separated integers into an array.
- Read the integer
- Calculate the Sum:
- Traverse the array and compute the total sum of its elements.
- Return the Result:
- Output the sum of the elements.
Simple Array Sum Problem Snippet
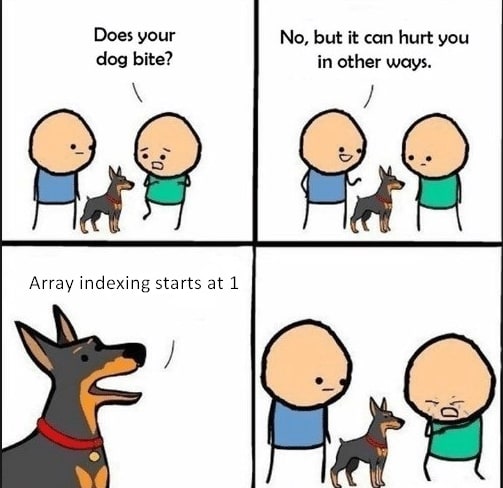
Simple Array Sum Solution
import java.io.*;
import java.math.*;
import java.security.*;
import java.text.*;
import java.util.*;
import java.util.concurrent.*;
import java.util.function.*;
import java.util.regex.*;
import java.util.stream.*;
import static java.util.stream.Collectors.joining;
import static java.util.stream.Collectors.toList;
class Result {
/*
* Complete the 'simpleArraySum' function below.
*
* The function should return an INTEGER as the result.
* The function accepts INTEGER_ARRAY ar as parameter.
*/
public static int simpleArraySum(List<Integer> ar) {
// Write your code here
int sum = 0;
for(int i:ar)
sum+=i;
return sum;
}
}
public class Solution {
public static void main(String[] args) throws IOException {
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bufferedWriter = new BufferedWriter(new FileWriter(System.getenv("OUTPUT_PATH")));
int arCount = Integer.parseInt(bufferedReader.readLine().trim());
List<Integer> ar = Stream.of(bufferedReader.readLine().replaceAll("\\s+$", "").split(" "))
.map(Integer::parseInt)
.collect(toList());
int result = Result.simpleArraySum(ar);
bufferedWriter.write(String.valueOf(result));
bufferedWriter.newLine();
bufferedReader.close();
bufferedWriter.close();
}
}
The Problem Solution