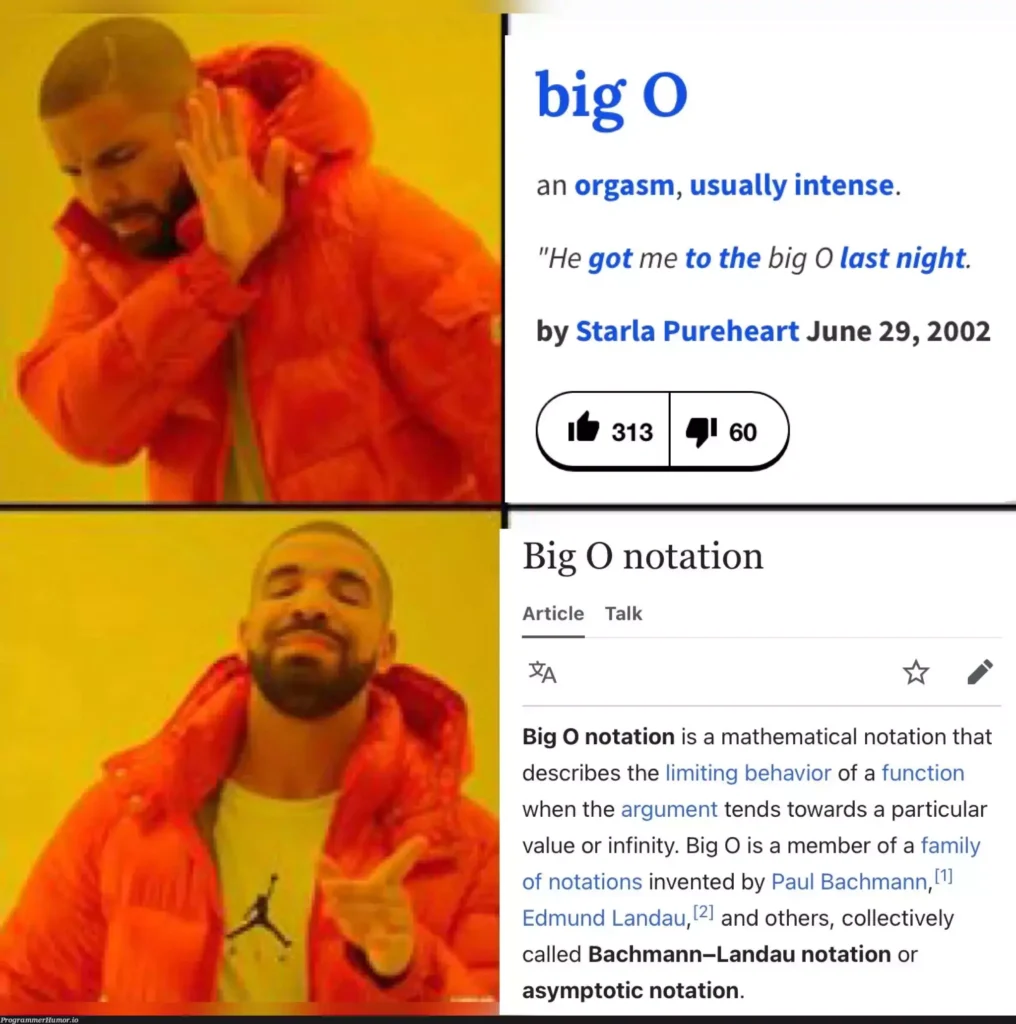
Overview
The ‘a very big sum‘ problem on HackerRank is a straightforward challenge where you must compute the sum of a large array of integers. The problem ensures that the sum of these integers can be huge, potentially requiring a 64-bit integer to handle.
A Very Big Sum Problem Statement:
You are given an array of integers and your task is to calculate the sum of all the elements. However, the challenge highlights that these integers can be huge, potentially exceeding the limits of standard integer data types in some programming languages.
Input Format
- The first line contains an integer n, which denotes the number of elements in the array.
- The second line consists of n space-separated integers that correspond to the elements of the array.
Output Format
Print the long integer sum of all elements in the array.
Example 1
Input:
5
1000000001 1000000002 1000000003 1000000004 1000000005
Output:
5000000015
Explanation:
The sum of the five elements in the array is 5000000015
.
Example 2
Input:
1
897685748769887678
Output:
897685748769887678
Explanation:
In this scenario, the array contains a single element, which is a very large number. The function correctly handles this case and returns the same value.
Key Points About ‘A Very Big Sum’:
- Large Integers: The prompt warns that the numbers might be very large, so you’ll need to use appropriate data types like
long
in C/C++/Java orlong
in Java to avoid integer overflow issues. - Sum Calculation: Iterate through the array, adding each element to a running total variable (
sum
) of the appropriate data type. - Return the Sum: After iterating through all elements, return the final
sum
value.
Remember:
- Choose the appropriate data type capable of handling large numbers.
- Implement a loop to iterate and accumulate the sum.
- Return the final sum.
A Very Big Sum Problem Snippet:
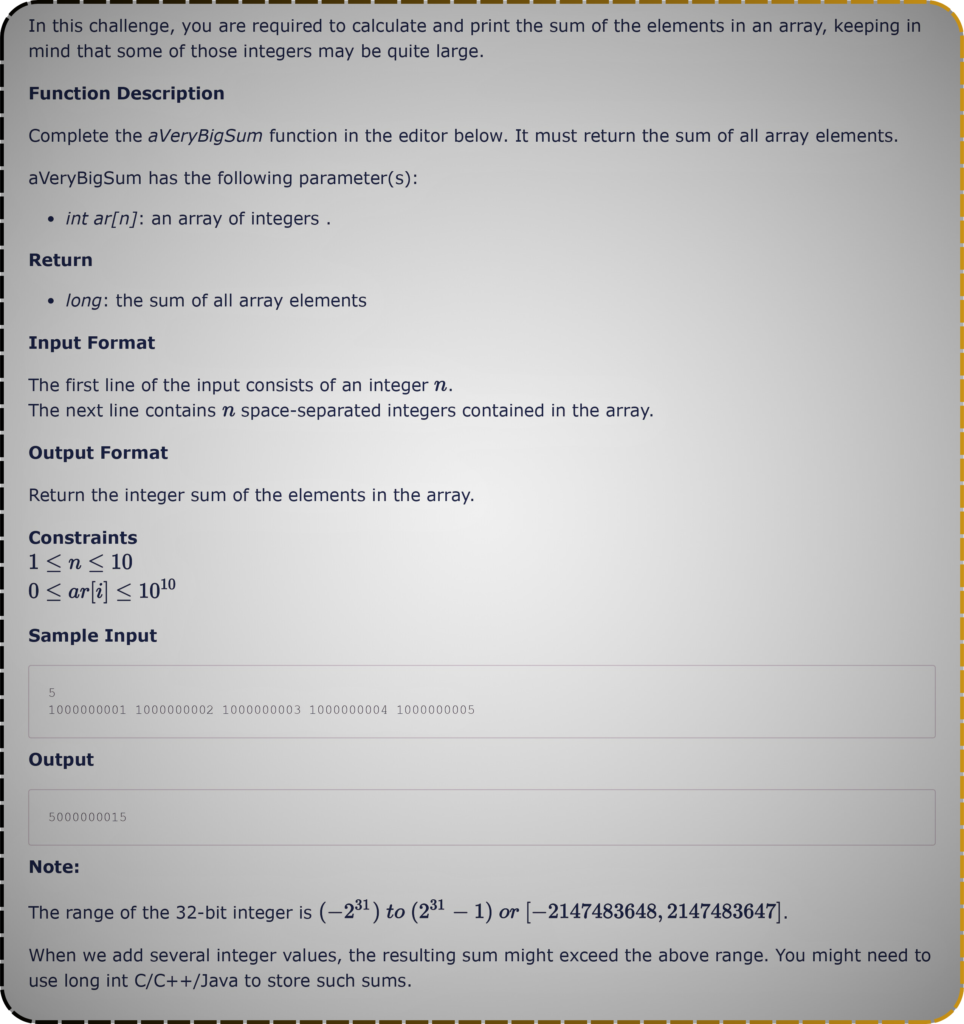
A Very Big Sum Solution:
import java.io.*;
import java.math.*;
import java.security.*;
import java.text.*;
import java.util.*;
import java.util.concurrent.*;
import java.util.function.*;
import java.util.regex.*;
import java.util.stream.*;
import static java.util.stream.Collectors.joining;
import static java.util.stream.Collectors.toList;
class Result {
/*
* Complete the 'aVeryBigSum' function below.
*
* The function is should return a LONG_INTEGER.
* The function accepts LONG_INTEGER_ARRAY ar as parameter.
*/
public static long aVeryBigSum(List<Long> ar) {
long sum = 0;
for(long l : ar)
sum += l;
return sum;
}
}
public class Solution {
public static void main(String[] args) throws IOException {
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bufferedWriter = new BufferedWriter(new FileWriter(System.getenv("OUTPUT_PATH")));
int arCount = Integer.parseInt(bufferedReader.readLine().trim());
List<Long> ar = Stream.of(bufferedReader.readLine().replaceAll("\\s+$", "").split(" "))
.map(Long::parseLong)
.collect(toList());
long result = Result.aVeryBigSum(ar);
bufferedWriter.write(String.valueOf(result));
bufferedWriter.newLine();
bufferedReader.close();
bufferedWriter.close();
}
}
The Problem Solution