A palindrome number is a number that remains unchanged even when its digits are reversed. To determine whether a number is a palindrome, we must compare the original number with its reversed version. If both are the same after reversing the number, the number is a palindrome.
For example, 121, 12321, and 1221 are palindrome numbers, while 123 and 1234 are not.
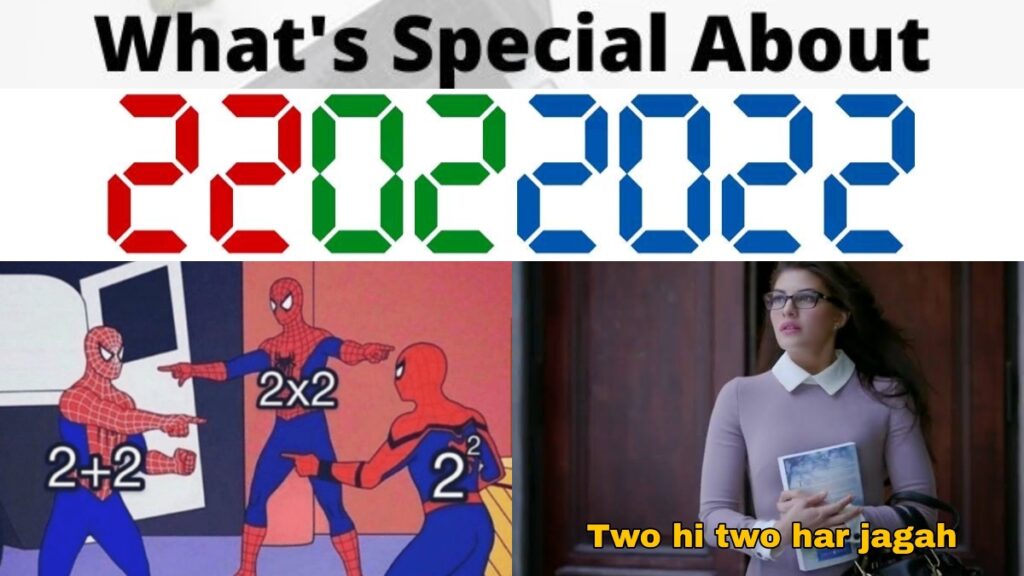
Table of Contents
Different Ways to Solve the Problem
1. Using StringBuilder Class
Steps:
- Convert the number to a StringBuilder
- Reverse the StringBuilder object by using inbuilt method reverse()
- Compare the reversed number with the original string using the parseInt() method.
public class PalindromeByStringBuilder {
public static boolean isPalindrome(int number) {
StringBuilder sb = new StringBuilder(number.toString());
return number = = Integer.parseInt(sb.reverse().toString());
}
public static void main(String[] args) {
int number = 121;
if (isPalindrome(number)) {
System.out.println("palindrome satisfied");
} else {
System.out.println("palindrome not satisfied");
}
}
}
The Problem Solution2. Using Mathematical Operations
Steps:
- Get the digits from the end of the number.
- Build the reversed number by appending these digits.
- Compare both the reversed and the original number.
public class PalindromeByMathematical {
public static boolean isPalindrome(int number) {
int original = number;
int reversed = 0;
while (number ! = 0) {
int digit = number % 10; // get the last digit by using mod(reminder)
reversed = reversed * 10 + digit; // building reversed number
number / = 10;
}
return original == reversed;
}
public static void main(String[] args) {
int number = 12321;
if (isPalindrome(number)) {
System.out.println("palindrome satisfied");
} else {
System.out.println("palindrome not satisfied");
}
}
}
The Problem Solution3. Using Recursive Call
Steps:
- Define a separate or helper function to reverse the number recursively by using mathematical operations.
- Compare both the reversed and the original number.
public class PalindromeByRecursiveCall {
public static boolean isPalindrome(int number) {
return number = = reverseNumber(number, 0);
}
private static int reverseNumber(int number, int reversed) {
if (number = = 0) {
return reversed;
}
int digit = number % 10;
reversed = reversed * 10 + digit;
return reverseNumber(number / 10, reversed);
}
public static void main(String[] args) {
int number = 54145;
if (isPalindrome(number)) {
System.out.println("palindrome satisfied");
} else {
System.out.println("palindrome not satisfied");
}
}
}
The Problem Solution4. Two-Pointer Approach
This approach is inspired by the two-pointer technique often used with arrays. You can treat the digits of the number as an array and check if the digits at the “start” and “end” are equal, moving inward.
Steps:
- Identify the first and last digits of the number.
- Use two pointers to compare the digits from both ends.
- If all digits match, the number is a palindrome.
Code:
public class PalindromeByTwoPointer {
public static boolean isPalindrome(int num) {
if (num < 0) return false;
int divisor = 1;
while (num / divisor >= 10) {
divisor *= 10;
}
while (num != 0) {
int firstDigit = num / divisor;
int lastDigit = num % 10;
if (firstDigit != lastDigit) {
return false;
}
num = (num % divisor) / 10;
divisor /= 100;
}
return true;
}
public static void main(String[] args) {
int number = 12321;
System.out.println(isPalindrome(number)); // Output: true
}
}
5. Using a Stack and Queue
You can push the digits of the number onto a stack and enqueue them in a queue. By comparing the stack and queue, you can determine if the number is a palindrome (since the stack is LIFO and the queue is FIFO).
Steps:
- Push each digit onto the stack and enqueue it into the queue.
- Pop from the stack and dequeue from the queue, comparing the values.
Code:
import java.util.LinkedList;
import java.util.Queue;
import java.util.Stack;
public class PalindromeByStackAndQueue {
public static boolean isPalindrome(int num) {
Stack<Integer> stack = new Stack<>();
Queue<Integer> queue = new LinkedList<>();
int temp = num;
while (temp > 0) {
int digit = temp % 10;
stack.push(digit);
queue.add(digit);
temp /= 10;
}
while (!stack.isEmpty()) {
if (stack.pop() != queue.remove()) {
return false; // If any digit doesn't match, it's not a palindrome
}
}
return true;
}
public static void main(String[] args) {
int number = 1221;
System.out.println(isPalindrome(number)); // Output: true
}
}
Overview and Comparison of Techniques
- StringBuilder Class:
- Pros: Simple and easy to understand.
- Cons: Involves converting numbers to strings, which can be less efficient for large numbers.
- Mathematical Operations:
- Pros: Efficient in terms of memory usage and works directly with numerical operations.
- Cons: Slightly more complex logic compared to string conversion.
- Recursive Call:
- Pros: Elegant solution using recursion.
- Cons: This can lead to stack overflow for very large numbers due to deep recursion.
Conclusion
Different techniques can be used to determine if a number is a palindrome in Java. The choice of technique depends on factors like simplicity, efficiency, and the context in which the solution will be used.